Building on the last blog, where we set up the LCD with an Arduino Wifi, we will now complete the project by connecting the Wifi, fetching the Bitcoin price, and displaying it on the LCD.
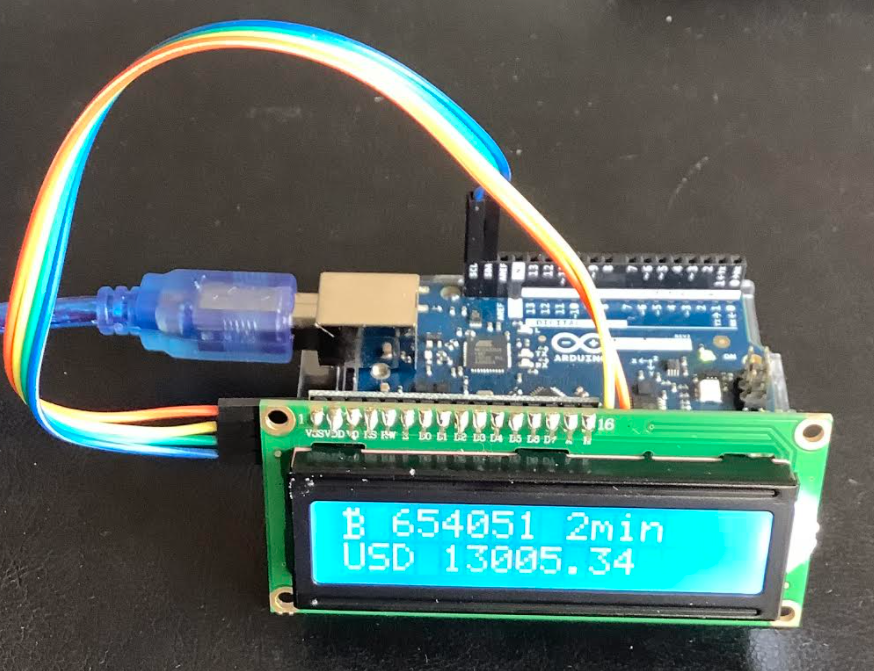
Updating the firmware
Install library WIFININA

Run the Firmware Updater, found in the tools menu
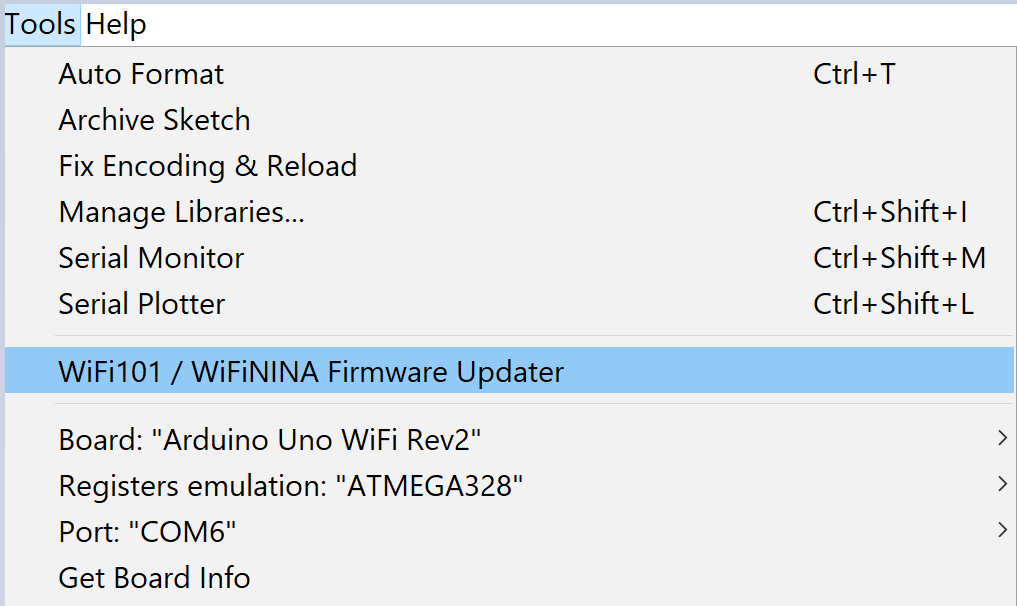
Test the programmer

Now run the Update Firmware, and then run the sketch CheckFirmwareVersion
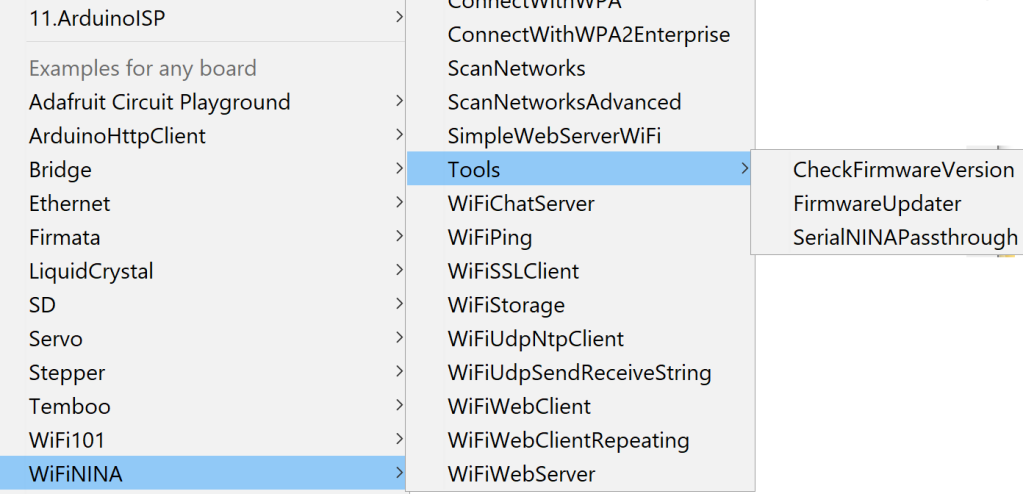
Notice that the checker still reports a newer version, in the Arduino forum it informs us that this can be ignored and in a future IDE version this will update correctly.
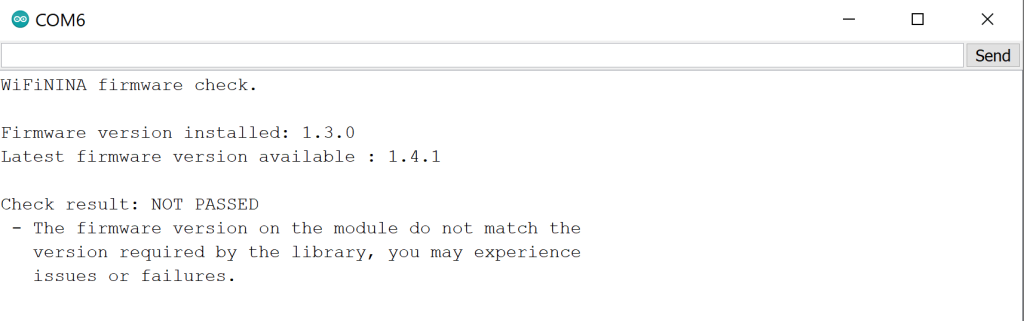
Install library on the Arduino IDE for a HTTP client

Code
Create a file in your project called arduino_secrets.h:
define SECRET_SSID "mySSID"
define SECRET_PASS "myPWD"
Creating the client with SSL transport
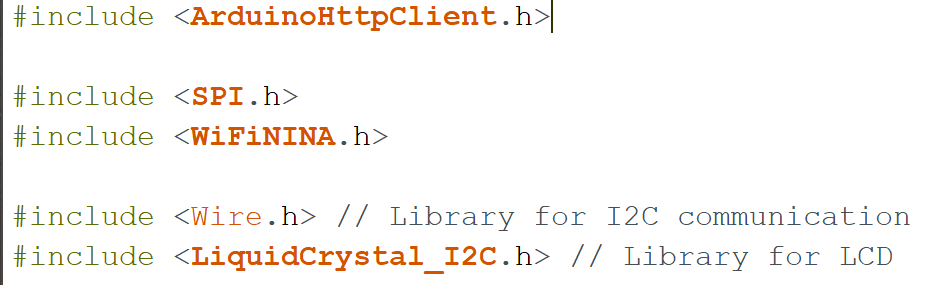
Using the following API from blochchain.info: https://www.blockchain.com/api/exchange_rates_api
char server[] = "blockchain.info";
int port = 443;
WiFiSSLClient wifi;
HttpClient client = HttpClient(wifi, server, port);
Making the HTTP request
In loop() {}
client.get("/tobtc?currency=USD&value=10000");
String response = client.responseBody();
btcPrice = 10000./response.toFloat();
// displaying on LCD
lcd.setCursor(0, 1);
lcd.print(btcPrice);
// add a delay
Upload the sketch and you should see the price updating.
Adding Bitcoin icon as custom character
Referencing this or using Excel:

byte Bitcoin[8] = {
0b01010,0b11110,0b01001,0b01001,0b01110,0b01001,0b01001,0b11110
};
...
void setup() {
...
lcd.createChar(0, Bitcoin);
...
lcd.write(byte(0));
That’s it for the moment. There are many ways to build on this setup, so be creative, and let me know in the comments below how you go.